In RxJS, a Pipeable Operator is essentially a pure function which takes one Observable as input and generates another Observable as output. Pipeable Operators return a new observable without modifying the original, inputted Observable.
What are Timing Operators?
Timing operators are a type of Pipeable Operators used to control when an Observable's values will be emitted. To control the flow of emitted values of an Observable, you can use debounceTime(), throttleTime(), sampleTime(), and auditTime() depending on the task at hand.
debounceTime()
debounceTime() emits the latest value after a pause/delay from when the latest value was emitted.
A popular use case for debounceTime() is to control when a user's input is "accepted" as to only take the last emitted value after a user pauses typing after a specified amount of time.
import { fromEvent } from 'rxjs';
import { debounceTime } from 'rxjs/operators';
const clicks = fromEvent(document, 'click');
const result = clicks.pipe(debounceTime(1000));
// Logs last value emitted after 1 second (1000 miliseconds)
result.subscribe(value => console.log(value));
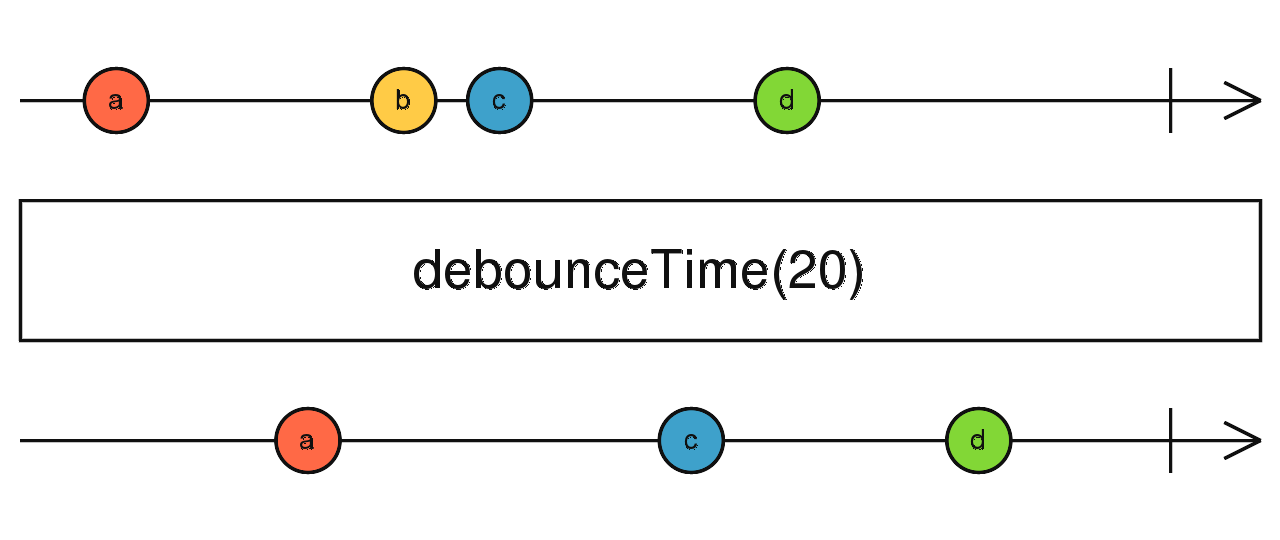
throttleTime()
throttleTime() is similar to debounceTime(). The difference is that throttleTime() emits a value first, then ignores values during a timed window and continues to repeat this pattern, whereas debounceTime() does not emit a value before the delayed windows start.
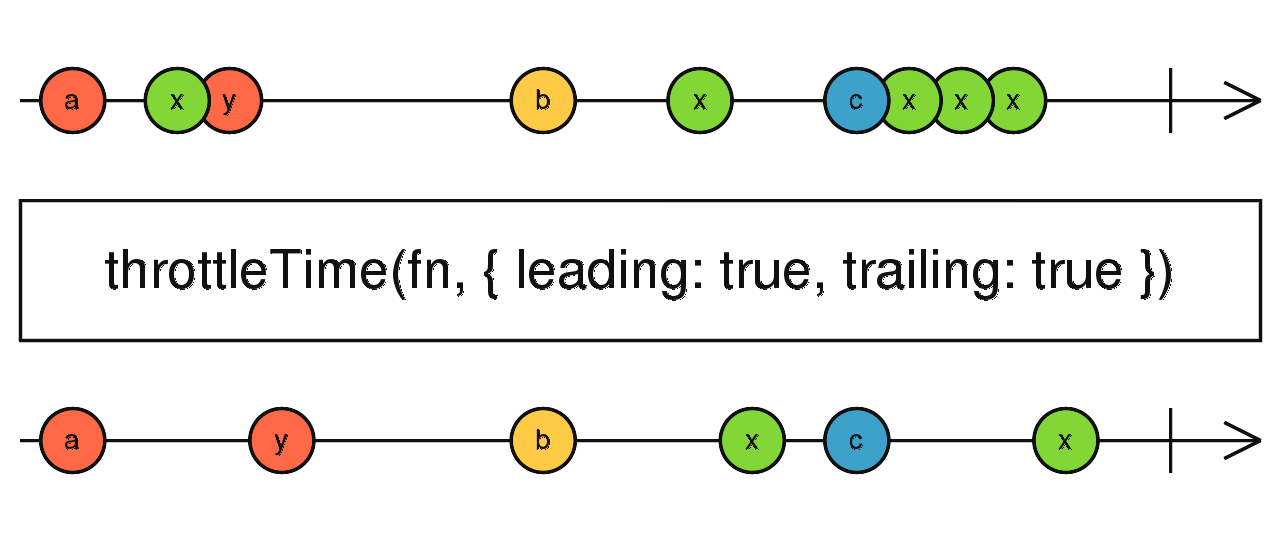
sampleTime()
sampleTime() emits the latest value at the end of each interval. Any earlier values are ignored, and silence window is repeated after subscription. Similarly, use sample() to sample an observable at any given time instead of a routine, preset interval.
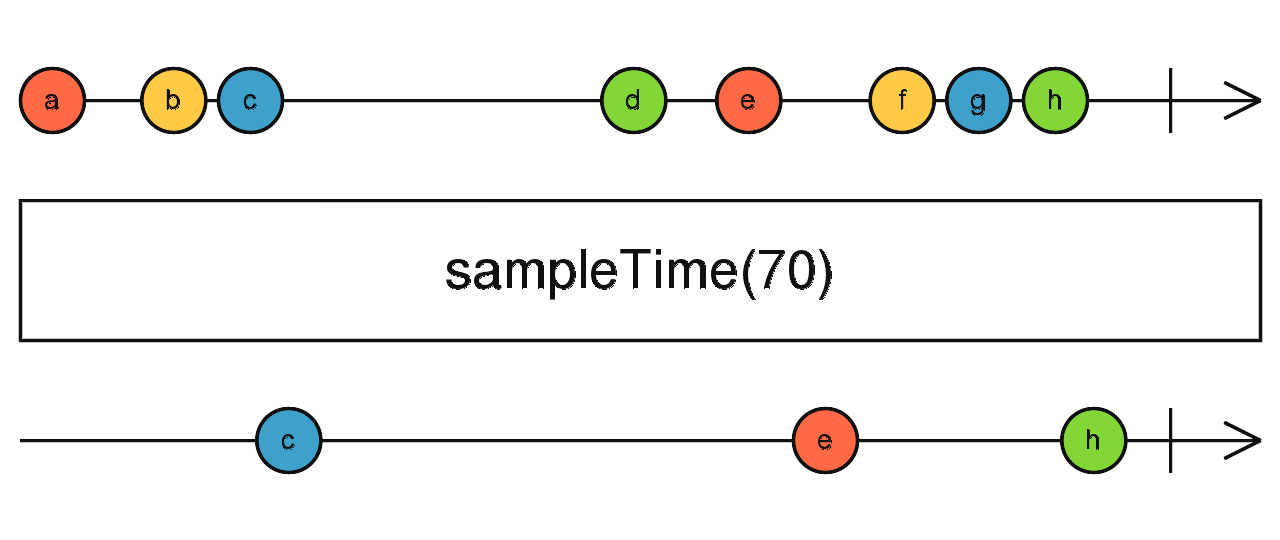
auditTime()
auditTime() ignores source values for the duration you specify after an emitted value. Silence window is triggered by emitted values from the source.
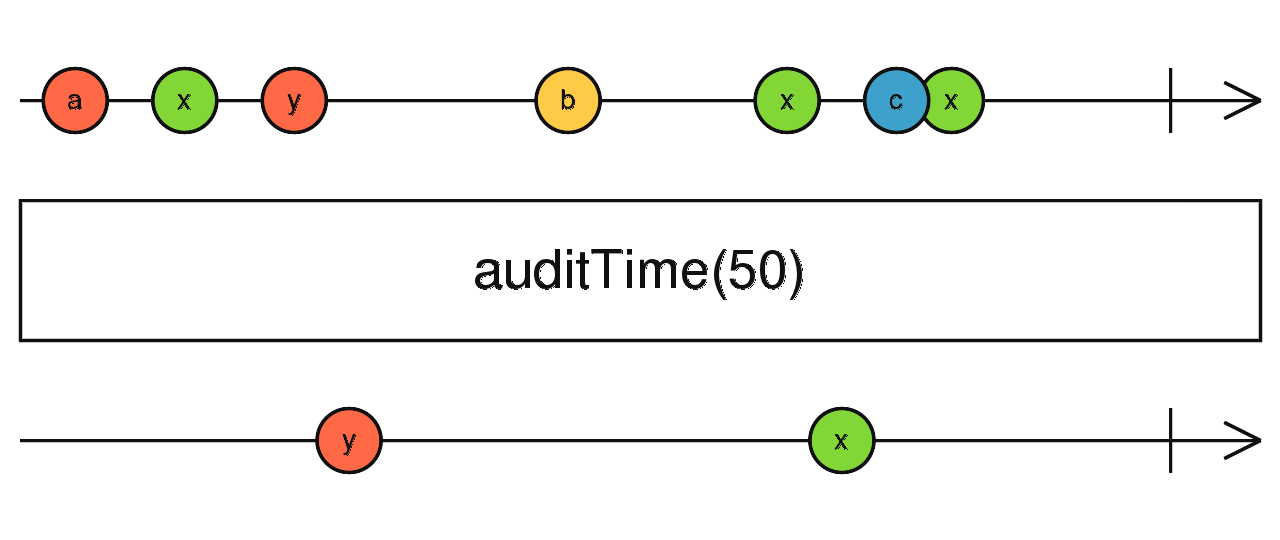
Conclusion
There are many ways to control the flow of emitted values of an observable, including filtering pipes and timing pipes. Depending on the timing behavior you need, take a look at debounceTime(), throttleTime(), sampleTime(), and auditTime() to see if these behaviors match what you are looking for. If none of these work for you, check out the other RxJS operators available.
Hope you enjoyed this article and of course feel free to reach out with additional questions. Be sure to follow us on Twitter to continue learning RxJS and subscribe to our blog for more amazing content.